String is an integral part of C++ programming and any other code. Explore the common functions with examples explained by trainer from a top C++ computer institute in Delhi, ESS Institute
What is string in C++?
The string is defined as a basic C++ data type in programming that stores a sequence of variable-length characters. There is another data type that can store characters and that is the “character” data type which we represent by ‘char.’ It can hold just one character at a time but then there may be times when we want to store a word or a sentence and such variable-length characters. In those cases, we will be making use of the string type which will come in handy.
How to initialise a string? How to declare string variable?
When we are using the <string> type, it is present under the <string> header and it is also available in the <iostream> header which we include in all of our programs. It is okay if you just include the <iostream> but it is a good practice if you explicitly include the <string> type.
You can do it either way:
#include <string>
Using std::string;
#include <iostream>
Using namespace std;
‘C-Style’ string and ‘std::string’ class:
C++ programming language offers two different types of string representations.
For example: “HELLO”
char name_of_array [10]; // declaration of string.For example: “HELLO”
char name_of_array [10]; // declaration of string.
When the value Is assigned to a string during its declaration, it is called string initialization.
Example:
char str [50] = “HELLO”;
char str [] = "Hello"; //stored as {'H', 'e', 'l', 'l', 'o', '\0'}

This is the traditional way of declaring strings in C++, where strings are represented as arrays of characters terminated by a null character.
Note: Always make the array one dimension longer than the string while working with C-strings. A c-string is referred to as a null terminator. If the length of the character is 5, then do not forget to make extra room for the null character.
The null character “\0” will be automatically added to the end of the string.
‘std::string’ class
The ‘std::string’ class is the most recommended way to handle strings in C++ because of its flexibility, efficiency, and safety. It does not need any null terminator to mark the end of the string. ‘std::string’ is more complex and managed which internally keeps track of the ‘size’ or ‘length’ of the string object.
For example:
#include <string>
std::string str1 = "Hello, World!"; // Declare and initialize a string
std::string str2;
str2 = "Welcome"; // Assign a value to the string.
Common String Function in C++
1. length() or size() – This Returns the length of the string that you specify. It returns the number of characters in the strings including all the spaces.
For example:
string message("Good morning!");
cout << message.length();
Output: 13
2. empty () – It returns ‘true’ if the string is empty(contains no character) and ‘False’ if not.
Syntax of using empty ():
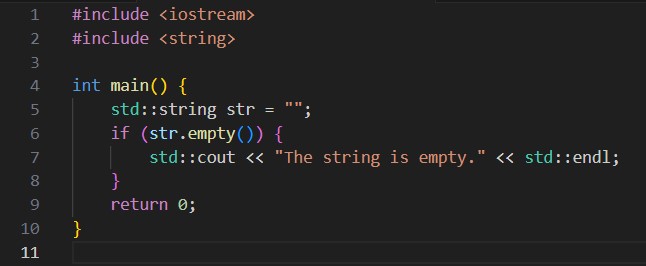
Output: The string is empty
3. clear () – This function removes all the characters from the string. It is useful when you need to reset something. The syntax we use to write this function is as below.
std::string str = "Hello";
str.clear(); // “Now str is an empty string "
Here is a sample code for the same:
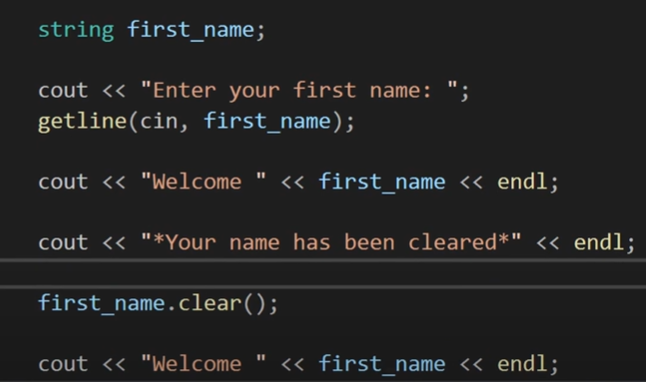
Output
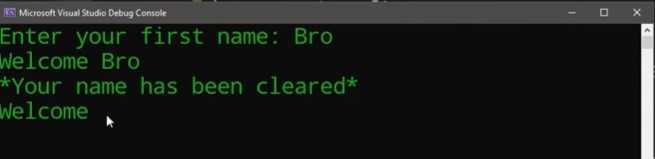
You can use this function when you need to clear out a form or any user input.
4. append () or + operator – This function will help you to add one string to another string. In other words, append () adds characters to the end of a string, and ‘+’ is used to concatenate two strings or a string with a character.
Syntax using append () operator:
std::string str1 = "Hello";
std::string str2 = " World";
str1.append(str2);
str1 is now "Hello World"
Syntax using ‘+’operator: Concatenation of two or more strings.
std::string str1 = "Hello";
std::string str2 = " World";
std::string result = str1 + str2; // result is "Hello World"
This example concatenates the strings str1 and str2. The result, “Hello World” is then assigned to the result.
For example: Imagine you are prompted for a username and we can actually append () an e-mail extension at the end to create an email address.
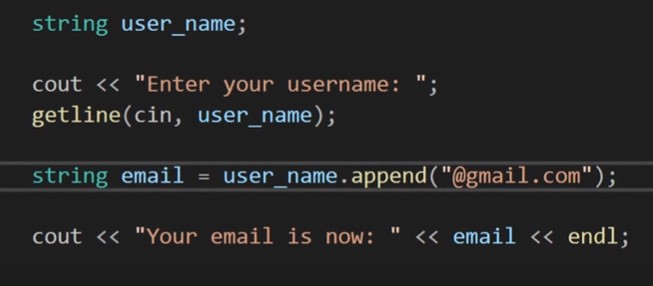
Output

5. substr () – This function returns a portion of the original string beginning at the specified position and going up to the length specified in characters from the starting position.
Syntax:
std::string str = "Hello, World!";
std::string substr = str.substr(7, 5); // This means starting from the 7thIndex i.e. ‘W’ and extract next 5 characters. In this case extracted substring will be ‘World.’

6. find () – Here, you can search for a substring inside a string and it will return the first instance of that substring or character that you have specified. It will return the position of the first character of a substring in an integer value or it will return a “std::string::npos” if the substring is not found.
Syntax and Example:
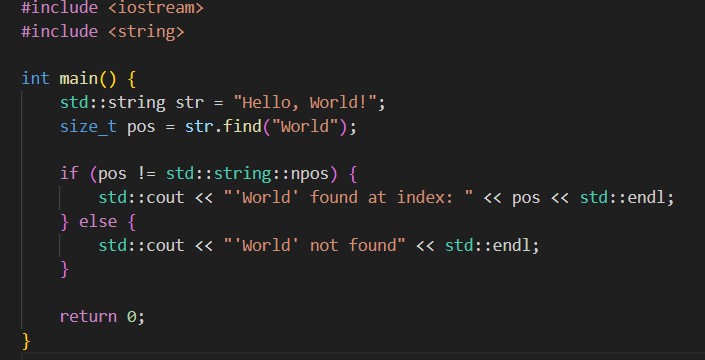
Output:
‘World’ found at index: 7
Modifying Strings in C++
The string class contains numerous methods for performing string manipulations such as inserting, erasing, and replacing.
- replace() – The replace() string is used to replace a part of a string with another string or characters. Here, we need to pass 3 arguments as follows:
Syntax:
str.replace(7, 5, "XYZ");
First argument: Starting position of replacement
Second argument: Length of string to be replaced
Third argument: The replacement String
For example:
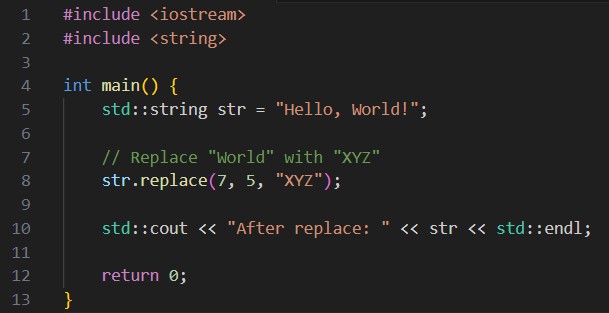
Output: After replace HELLO, XYZ!
2. insert() – The method insert() inserts a string at a certain position of another string. The position is passed as the first argument and it will define the character before which to insert the string.
Note: The first character in a string occupies position 0, the second character position 1, and so on.
For example:
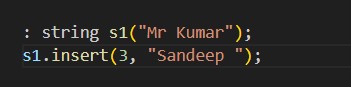
The string “Sandeep ” is inserted into the string s1 at position 3, which is in front of the ‘k’ character in “Kumar”. Following this, the string “Mr Sandeep Kumar ” is assigned to s1.
3. erase() – You can use the erase() method to delete a given number of characters from a string.
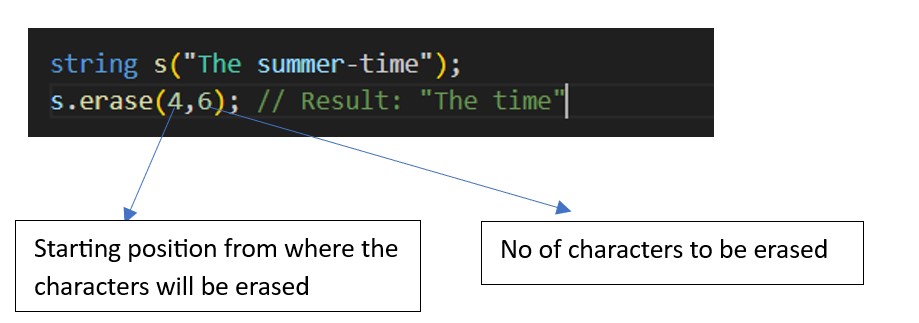
This statement deletes 7 characters from string ‘s’ starting at position 4. The erase() method can also be called without specifying a length and will then delete all the characters in the string up to the end of the string.
String Comparison in C++
- compare(): The compare() function is used to compare an original string to another string or a substring. It returns 0 if the strings are equal, a negative value if string 1 is smaller than string 2, and a positive value if string 1 is greater than string 2.
Syntax:
int compare(const std::string& str) const;
This is for the comparison of string1 with string2.
For Example:
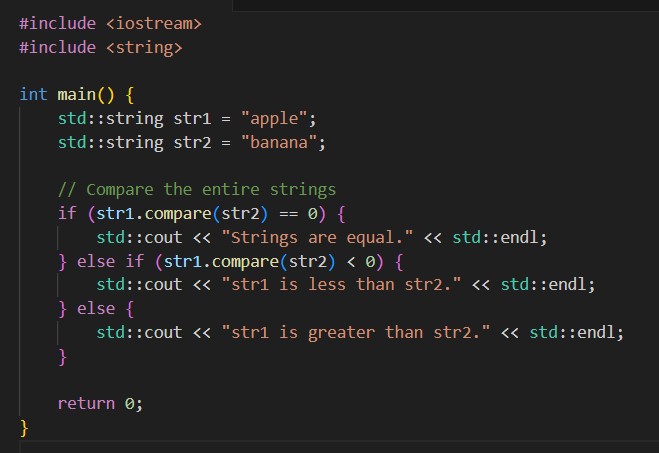
Output: str1 is less than str2
In C++, the standard class string, which is used to represent strings provides us with various functions to perform on strings such as searching, comparison, concatenation, string modification, and utility functions such as empty(), clear(), and Substr(). We have covered it all in the above topics with some instances. You can also reach out to our skilled mentors in ESS INSTITUTE, DWARKA if you want to learn C++ in Delhi and other programing language like python in more depth. While developing yourself with our team, you will be getting plenty of resources and hands-on some real-world problems in a much more effective way. Quickly, check out our website to see the list of courses you are willing to join.