Object-Oriented Programming, as the name makes clear, uses objects in programming. It is a style of programming that models and arranges code using objects and classes. Real-world concepts like inheritance, hiding, polymorphism, etc. are intended to be incorporated into programming through Object-Oriented Programming.
In this artcle, C++ trainer from a leading C++ Institute in Delhi will explain the OOP concepts in easy ways
Programming languages like C++ are flexible and fully support OOP concepts.The core objective of OOP is to bind together the data and the functions that use them such that only that function and no other section of the code can access the data.
OOPs provide a number of advantages for both users and designers, and they are crucial in many fields, such as user interface design, simulation, modeling, etc. The article on C++ OOPs will help you comprehend and learn all there is to know about C++ Object-Oriented Programming.
Object-Oriented Programming: Why Do You Need It?
There were various drawbacks with the early methods of programming as well as poor performance. The technique couldn’t tackle real-world issues very effectively since, similar to procedural-oriented programming, global data access was a challenge and code couldn’t be reused within the program.
Whereas in OOPs,
- Classes and objects make it simple to maintain the code.
- The program becomes more simple as inheritance allows for code reuse, which prevents you from having to write the same code repeatedly.
- It also makes data concealing possible with concepts like encapsulation and abstraction.
Basic Concepts of Object-Oriented Programming (OOP) in C++
OOP is centered on a few fundamental concepts that serve as its building blocks. They are:
- Classes & Objects
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
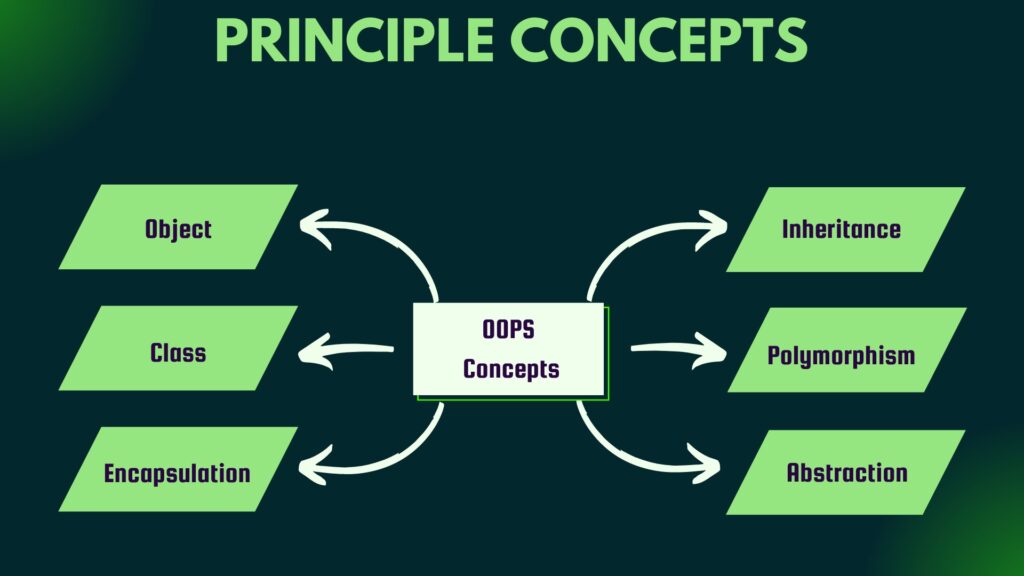
Let’s explore the aforementioned concepts using C++ examples and applications.
Classes & Objects
An object is everything that physically exists in the world and has a state and behavior, or, to put it another way, anything that has a name. It could be used to depict a table, a dog, or a human. An object is a collection of information and programming that further represents an entity.The programming language C++ is object-oriented.
In C++, everything is related with classes and objects, as well as their characteristics and functions. An automobile, for example, is an item in real life. The automobile contains characteristics like weight and color, as well as functions like drive and brake.
A Class is a blueprint for an object. A Class in C++ is the foundational element that leads to Object-Oriented Programming. It is a user-defined data type that can be accessed and used by creating an instance of that class. It has its own data members and member functions.
Consider the class of cars, for instance. There can be numerous automobiles with various names and brands, but they all have some characteristics, such as having four wheels, having a speed limit, having a range in miles, etc. In this case, the Car is the class, and its attributes include its wheels, speed limitations, and mileage.

- Having both data members and member functions, a class is a user-defined data type.
- Together, data members and member functions describe the properties and behaviors of the objects in a Class. Data members are the data variables, and member functions are the functions used to control these variables.
- In the previously mentioned car class example, the speed limit, mileage, etc., will be data members, and member functions will be brakes, speed up, etc.
Abstraction
One of the most crucial components of C++ object-oriented programming is data abstraction. Data abstraction is the process of exposing to the outside world just the information that is absolutely necessary while concealing functionality or background information. It avoids providing extraneous or unnecessary facts and only displays the precise portion the user has requested.
Take a look at a man operating a vehicle in the real world. The man only knows that hitting the accelerator will make the car go faster and that applying the brakes will make the car stop, but he has no idea how pressing the accelerator actually increases the speed of the car. He also has no idea how an accelerator, brakes, etc. are used in the vehicle. Abstraction is exactly what it is.
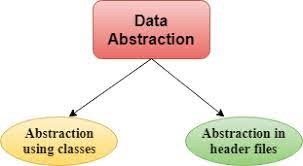
- Abstraction using Classes: We can use classes to implement Abstraction in C++. Using the available access specifiers, the class enables us to organize data members and member techniques. Which data member is visible to the public and which is not can be determined by a class.
- Abstraction in Header files: Header files are yet another type of abstraction in C++, such as the pow() method found in the math.h header file. Whenever we need to calculate the power of a number, we just use the function found in the math.h header file and pass the numbers as arguments without knowing the underlying algorithm that the function is actually using to calculate the power of numbers.
Abstraction has the following benefits;
- Abstraction hides implementation details, exposing a user-friendly, simplified interface with less complexity that enables interaction with high-level abstractions without being aware of the complexity at the foundational level.
- By concentrating on important features and obscuring unimportant details, abstraction improves code maintainability by minimizing the effects of changes to the underlying implementation on other codebase components.
- Abstraction enhances flexibility and enables code adaption without changing the original code by enabling developers to construct universal interfaces for a variety of use cases.
1. Encapsulation
Encapsulation is the process of combining data and functions into a single entity. It makes sure that people cannot see “sensitive” info.The use of access specifiers like public, private, and protected helps to enforce encapsulation in C++.
To gain access to these data members, make the scope of the data members private and the scope of the member function public.You can use public get and set methods if you want people to read or edit the value of a private member.
Following are a few advantages of Encapsulation:
- Encapsulation safeguards an object’s internal implementation details, preventing unauthorized access or alteration and assuring data integrity and state consistency.
- Encapsulation improves code organization by grouping similar data and methods into a class, reducing code clutter and enhancing readability and maintainability.
- Encapsulation fosters code reuse by enclosing behavior within objects. It is possible to reuse objects across several projects or portions of the code, which promotes effective development and reduces repetitive code.
2. Inheritance
Inheritance is the process through which objects belonging to one class acquire the traits and attributes of the other class through an is-a relationship between the two classes.Inheritance is an essential aspect of Object-Oriented Programming.The “inheritance concept” is segmented into two categories:
- Sub Class/ Derived Class (Child): the class that inherits from another
- Super Class/ Base Class (Parent): the class being inherited from
Use the “:” sign to indicate that you are inheriting from a class.
Example: Cars, Bikes & Buses can be Derived Class of Vehicle Class.
Some of the advantages of Inheritance:
- Code reuse is promoted through inheritance, allowing classes to inherit traits and behaviors from base classes, enhancing and specializing functionality without the need for redoing common code.
- Polymorphism is made possible via inheritance, enabling objects of derived classes to be considered as objects of their base class, encouraging flexibility and dynamic programming.
- Using a systematic representation of relationships and dependencies, inheritance establishes hierarchical links across classes, improving the organization, comprehension, and maintainability of the code.
3. Polymorphism
When there are several classes that are connected to one another via inheritance, a phenomenon known as polymorphism—which means “many forms”—occurs. As stated in the headline above, inheritance enables us to take characteristics and functions from another class. Polymorphism uses these approaches to carry out various objectives. This enables us to carry out a single activity in a variety of ways.
Consider a base class named Animal, which has the function animalSound(). Pigs, Cats, Dogs, and Birds are derived classes of animals that each have their own unique animal sound (the pig oinks, the cat meows, etc.).
Polymorphism allows many classes to be considered as instances of the same base class. It enables you to create code that interacts uniformly with objects belonging to various classes.
There are various benefits of Polymorphism:
- Polymorphism allows objects to be treated uniformly via a single interface, increasing code flexibility by allowing functions or algorithms to interact with multiple kinds without requiring particular implementations.
- Polymorphism enables the introduction of new derived classes that align with the same interface without altering the current code, thereby reducing maintenance and facilitating new features.
- Polymorphism increases code readability by expressing purpose and behavior through abstract interfaces, allowing for more succinct and expressive code that focuses on the task at hand.
Hopefully, the purpose of Object-Oriented Programming, what C++ OOP are, and the fundamental OOP ideas like polymorphism, inheritance, encapsulation, etc. should all be clear to you now that you’ve read this article. Along with polymorphism and inheritance examples, you also learnt about the benefits of OOP.
You can also attend our offline classes in our C++ institue in Dwarka for more detailed learning experience