The intricate world of memory allocation, where data is stored and accessible within a computer’s memory, is hidden behind the scenes of every programme. Memory management is automatically handled by the interpreter in the realm of Python, a potent high-level programming language, relieving developers of the necessity of explicit memory allocation. However, a deeper knowledge of memory optimization and programme speed may be unlocked by grasping the ideas of dynamic and static memory allocation. We’ll take a voyage through Python’s remarkable memory allocation system in this engrossing blog article, studying the distinctive strategies of dynamic and static allocation. Here we will unravel the secrets and ramifications of memory allocation, revealing knowledge that will enable you to create Python code that is both efficient and reliable.
For some students or professional python programmers, memory allocation in Python is still a confusing concept hence to help them get the carity, Our top python instructors from Best Python Institute in Delhi explains the concept with example
Static Memory Allocation in Python
Static memory allocation refers to declaring fixed-size data structures or allocating memory for data structures at the time of compilation. Even though Python doesn’t explicitly allocate static memory, there are some situations in which static-like behavior is preferred. Let’s investigate these situations:
Example
def static_memory_allocation():
# Create a fixed-size tuple
my_tuple = (1, 2, 3, 4, 5)
# Print the tuple
print("Tuple with static-like memory allocation:")
print(my_tuple)
# Attempt to modify the tuple (this will raise an error)
try:
my_tuple[0] = 10
except TypeError as e:
print(f"Error: {e}")
static_memory_allocation()

Immutable Things
Strings, tuples, and frozensets are examples of immutable objects in Python that behave similarly to static memory allocation. Once they’ve been created, their values can’t be changed. Python reduces memory use by recycling immutable objects as often as feasible. For instance, if you make two strings with the same value, they will both refer to the same location in memory and use less memory.
Global and Constant Variables
In Python, global variables and constants are examples of static memory allocation. Memory is allocated to hold the value of a constant or global variable when you define one, and that memory address is fixed for the duration of the programme. Programme behavior is consistent and predictable because to this fixed memory allocation.
Functions using Static Variables
Although static variables within functions aren’t explicitly supported in Python, you may nevertheless get comparable results by utilizing closures or class properties. Variables that are defined within a function’s scope keep their values after function calls. Within function contexts, this approach enables a kind of static memory allocation.
Dynamic Memory Allocation in Python
By allocating memory at runtime, dynamic memory allocation offers flexibility in memory utilization. Dynamic memory allocation in Python usually works through making use of pre-built data structures like lists, dictionaries, and objects.
Example
def dynamic_memory_allocation():
# Create an empty list
my_list = []
# Add elements to the list dynamically
for i in range(1, 6):
my_list.append(i)
# Print the list
print("List after dynamic allocation:")
print(my_list)
# Remove an element from the list dynamically
my_list.remove(3)
# Print the list after removing an element
print("List after removing an element:")
print(my_list)
dynamic_memory_allocation()
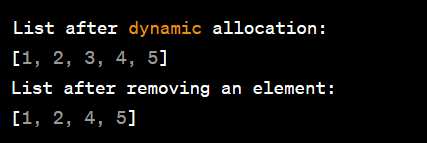
Let’s explore dynamic memory allocation in more detail:
Dynamic Lists
Python lists are dynamically allocated and can expand or contract as necessary. A block of memory is allocated to accommodate the list’s initial size when it is initialized. If more space is needed for the list to grow, new memory is allocated and the current components are copied over to it. Large datasets may be handled and effective memory use is ensured by this dynamic scaling.
Dynamic Objects
User-defined classes and other Python objects are dynamically allocated in memory. Each object comes with a header that identifies the type and reference count of the object, followed by the object’s contents. Memory is allotted when you create an object to hold its characteristics and methods. When objects are no longer referenced, Python’s garbage collection function automatically frees up memory, enabling memory management easier for developers.
Dynamic Memory
Management: Automatic memory management of Python uses reference counting and garbage collection mechanisms. Reference counting counts how many times an object is referenced and deallocates memory when the count is zero. Garbage collection identifies and recovers unreachable objects in situations where circular references prevent objects from being deallocated.
Programme Performance and Considerations
Memory use and programme performance are impacted by both dynamic and static memory allocation mechanisms. When deciding between them, take into account the following factors:
- Dynamic memory allocation offers flexibility by enabling data structures to expand or contract as necessary. When working with different data quantities or user-generated material, this flexibility ensures effective memory utilization. However, because of memory resizing activities, dynamic allocation has overhead. Through the allocation of a predetermined amount of memory at compile time, static memory allocation enables efficiency. Resizing procedures are no longer necessary, which leads to predictable memory consumption patterns and perhaps speedier programme execution. However, if the assigned size is more than the actual required, static allocation may result in RAM waste.
- Memory fragmentation, in which memory is gradually fragmented into small, non-contiguous pieces, may be brought on by dynamic memory allocation. Performance in memory deallocation and allocation can be affected by fragmentation. Memory compaction and memory pools are two methods that might lessen fragmentation problems.
- Memory Control, For developers, memory allocation and deallocation are made simple by Python’s automated memory management. When objects are no longer being referred, the reference counting technique assures prompt memory deallocation. Instances where circular references prohibit objects from being deallocated are handled via garbage collection. These methods, nevertheless, come with some extra computational work.
- Enhancing Memory Usage, There are methods to optimize memory utilization in Python regardless of the memory allocation strategy. Programme speed may be improved by using methods like object reuse, iterators, generators, memory views, and context managers. Your Python programmes can utilize memory effectively if you comprehend and use these approaches.
Winding up
Programming relies heavily on memory allocation, and knowing how dynamic and static memory allocation work in Python help with memory optimization and programme speed. The allocation and deallocation operations are made simple by Python’s automated memory management, allowing developers to concentrate on the logic of their applications. You may develop Python code that maximizes memory utilization and improves overall programme performance by taking into account the trade-offs between flexibility and efficiency, comprehending memory fragmentation, and using memory optimization techniques.
You can also join our python course institute in Delhi to learn python like a pro.