As the name suggests, AngularJS is JavaScript based framework. AngularJS nowadays has been evolved as a technology that allows us to create the client-side web application using JavaScript. This simply means that our websites will be efficient enough to deliver faster output.
Download Angular from here
What is AngularJS?
Before getting started, let us look on the prerequisites:
- You should ensure familiarity with HTML and CSS to create your own HTML web pages. Once you are done with that you can Implement AngularJS in the same HTML pages.
- To write the AngularJS code, you should also have the sufficient knowledge of JavaScript.
- Good knowledge of JavaScript will be providing you an edge in implementation.
Web development technology is a widely used approach to create distributed applications. A distributed application is one where the application is deployed on a single machine but can be accessed by remote clients. This has always been one of the most cost-effective ways to build distributed applications.
Why AngularJS is Perfect for Modern Web Development?
However, a common issue associated with web development is page-posting. Page-posting occurs when a web page request is sent to the server and then returned, a process known as postback. This communication between the client and server consumes significant time, making web applications less efficient compared to Windows applications.
So, considering the same fact, Adam Abron’s and Miško Hevery in 2009 brings the logic of a framework called AngularJS. In a very short span of time, this technology become popular among the organizations and the companies because it is an open-source and completely free. Any type of organization any developer can use the angular JS in their application to make the application faster without paying any extra cost.
It is licensed under Apache and it is maintained by Google. When we will set the environment variable of AngularJS, we will see the participation of Google in that.
Using AngularJS, you are good to create the RIA application, where RIA stands for Rich internet applications but apart from that the best feature of AngularJS which we felt so far is the inclusion of MVC architecture. MVC architecture nowadays is so popular that so many vendors like Microsoft, Java are all using this architecture to create their web applications. But here, we can implement the same architecture in our AngularJS so that it will give you even faster application because everything is done in JavaScript without any post back.
Apart from that, AngularJS is compatible with all the available latest version of browsers like Google Chrome, Mozilla Firefox, and Internet Explorer.
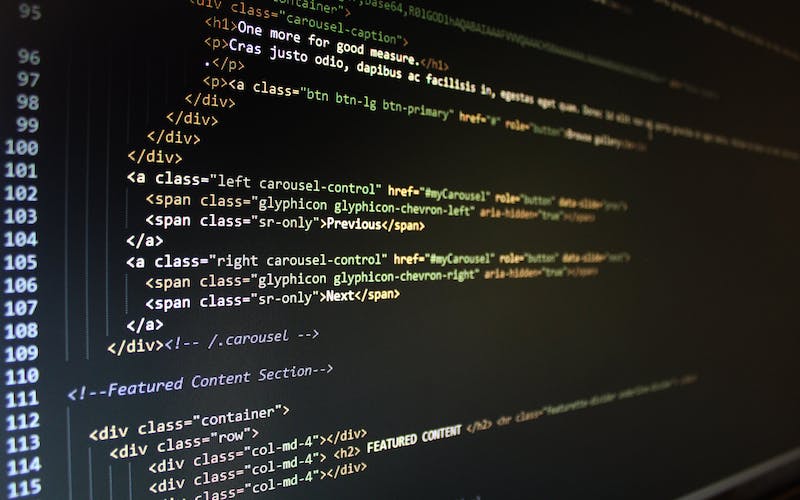
Key features of AngularJS
- SPA: SPA- stands for Single Page Application. SPA allows different views to be loaded into a shell page as user interacts with page. SPA maintains history of views that have been displayed so if you will click on the back button it will go back to previous view. Normally when you click on the back button, you will go back to previous website.
- Two-way Data-Binding: Two-way Data binding always keep model and view updated at same time. If there is any change in model, it will automatically update view. Similarly, when the view has any changes, it updates model respectively.
- Dependency Injection: Dependency Injection is a software design pattern that deals with how objects and functions get created and how they get a hold of their dependencies.Everything with Angular (directive, filters, controller, services) is created and wired using Dependency Injection.
- Directives: Directives are the most important component of any AngularJS application. There are many directives available in AngularJS. These are the markers on DOM element which attach special behaviour to them.
- Test-Driven: AngularJS shines in Testing and this is where AngularJS has upper hand. With AngularJS unit testing and end-to-end testing has become easier.
When we first time started learning AngularJS, it felt very overwhelming to see lot of parts available and struggled to understand how to fit them together. So according to us, easiest way to learn AngularJS is to know what are the different parts available, how different parts fit together and how we can organise different parts to actually make full application. So, when you look different parts in big picture view it will look something like this:
So, on the very top we have something called Modules.
Modules in AngularJS
- Modules act like container that is used to hold components that you will create like controllers, directives, filters, services and factories.
- Routes: This is how we will define which view we need to load. Route is a path which you see in browser. After route there are two categories in which you can divide this concept.
- The first one is UI side and the other one is logic/data.
- View is actually a web page but it also needs data to have some logic. So, on the UI side we have views, and in the view, we can have directives which enhances HTML.
- Filter: This provides some data filtration functionality.
- On the logic/data side we have controllers: It acts like a brain for our views and controller can have data from factories and services. Once we have data in the controller, we need to pass this data to view and we do it by using scope.
- Scope act like a view-model that provides data to my view. It is like a glue between controller and view.
How to set-up AngularJS?
It needs 3 steps to get started with AngularJS:
- Reference the AngularJS script in html page.
- Add ng-app directive into your shell page.
- Bind data using directives.
How AngularJS works?
Step 1: Reference the AngularJS Script in your HTML page
Add a reference to a local version
<script src=”scripts/angular.js”></script>
After downloading the AngularJS library file and saving it on your computer, you will copy the exact path of the file and link it to your project using this line:
Add a Google CDN:
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.8/angular.min.js"></script>
CDN stands for Content Delivery Network. This allows you to use AngularJS without downloading it. The library is hosted on Google’s servers. This method ensures faster loading and easy updates since it’s served from a reliable source.
Step2: Adding ng-app Directive into HTML page
<!DOCTYPE html>
<html ng-app>
</html>
You can add ng-app directives to either the ‘html tag’ or the ‘body tag.’
The ng-app directive tells AngularJS where your application starts. By doing this, AngularJS knows to activate its features for everything inside the <html> tag. It will initialize your AngularJS application.
Step3: Bind Data using Directive
Use AngularJS directives to add data-binding functionality into a page:
Name: <input type=”text” ng-model=”name”/> {{name}}
<!DOCTYPE html>
<html lang="en" ng-app>
<head>
<meta charset="UTF-8">
<title>AngularJS Basics</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
</head>
<body>
<h1>Welcome to AngularJS</h1>
<p>Type your name below:</p>
<!-- Input Field for User Name -->
<input type="text" ng-model="name">
<!-- Display the User's Name Dynamically -->
<p>Hello, {{ name }}!</p>
</body>
</html>
Data binding in AngularJS allows you to connect the user interface (UI) with the data. This means changes in the input field are automatically reflected elsewhere on the page.
Here’s an example of an input field that allows users to type their names:
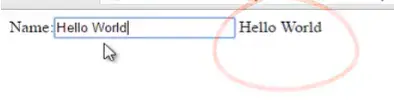
As you type your name, AngularJS stores it in a property of the model object. The model object is just an object that holds the data and we have named the property ‘name’. This ng-model here is a directive.
Next, we will bind this data to our view, using data binding expression. To do this we will go through some steps:
You need to put double curly brackets and then the ‘name of the property’ that you want to bind.
Here, we have defined a property called name using ng-model. You just need to put the ‘property name’ in the double curly brackets. Whatever user will type on the text box, it will also write in place of this data binding expression.
The ng-model=”name” connects the input field to a variable called name.
When the user types something into the input field, the value is instantly stored in name.
The {{name}} syntax displays the value of the name variable. It updates in real-time as the user types.
We have come so far and learnt a lot of core concepts for one who is thinking of starting up with AngularJS.
AngularJS is helpful in building interactive, dynamic, and scalable web applications with ease which makes it a top choice among this generation. If you still need any further assistance, or need to enrol in any web development course, you can reach out to our skilled mentors in ESS computer Mern stack Institute Delhi. Our team is constantly working to make things easier to learn for all of you. Get in touch with us if you really want to upskill yourself and widen employment options as well.
Keep learning